Decoding JavaScript Date Libraries: Find the Perfect Fit for Your Project
by Rui Fernandes -
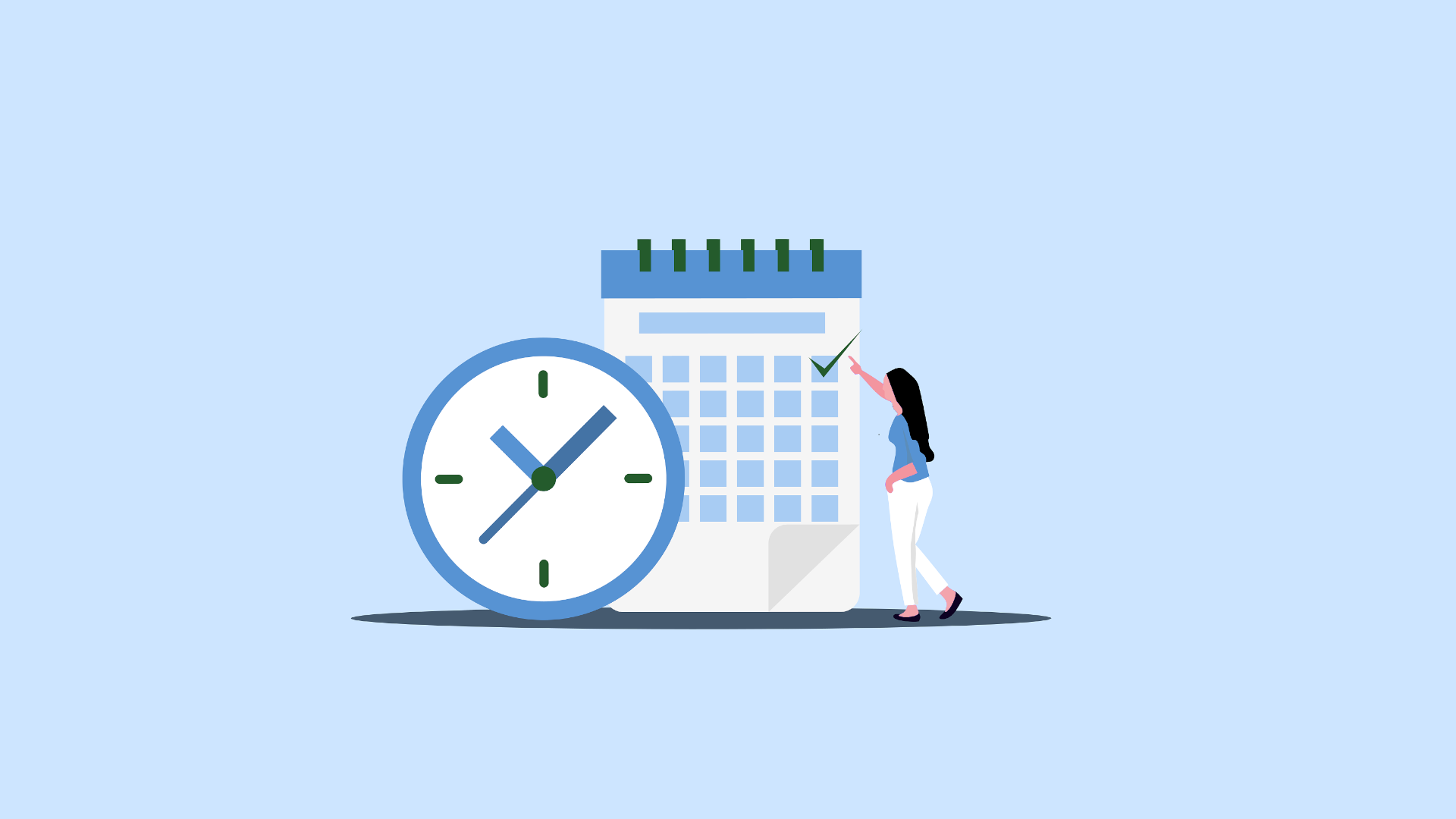
Introduction
Handling dates is crucial in any application, from displaying the current date to performing essential calculations for business rules. The process of dealing with dates can be summarized in four pillars:
- Conversion: Parsing different types of data, e.g., string to Date, Date to string.
- Formatting: Formatting a date in a specific pattern, e.g., YYYY-MM-DD.
- Transformation: Modifying the values of a date, e.g., adding 2 days.
- Difference: Obtaining the difference between dates, e.g., time remaining between the current date and a specific date.
- Comparison: Comparing instances of dates, e.g., determining if one date instance is ahead of another.
In JavaScript, this process becomes more challenging due to its outdated Date API.
To overcome this, some alternatives as the official Temporal.js API and various libraries have been created.
Unfortunately, Temporal.js is not ready to be used in production, as stated in its official documentation, so we need to use external libraries, such as Moment.js, Date-fns, Day.js and Luxon, which are notable alternatives. This article aims to compare these libraries, considering aspects such as maintenance, mutability, and other critical features.
Moment.js
Mutability
Moment.js operates on the mutability paradigm. Instances of Moment objects can be modified, leading to potential side effects, as demonstrated in the provided example:
In [1], we add 3 days and assign the result to the variable someDate. Notice that this addition operation not only returns an object X (remember this name, as we'll explain this object later) but also modifies the original object, which is in the variable current.
Considering this mutability, in [2], we add another 4 days to the someDate object using the add() method. Indeed, the someDate object was updated without the need for reassignment. However, this add() method call caused a side effect on current.
Thus, we can conclude that, in reality, the object X that the add() method supposedly returned is not a new object but a pointer referencing the current variable in memory. In other words, any changes made to someDate are reflected in current.
One way to workaround this situation is by using the clone() method, but it incurs a higher computational cost than simply returning a specific value or a new object.
In this context, the library maintainers do not intend to make the switch to an immutability methodology. This decision is based on the fact that it would completely disrupt the current library, while other libraries already successfully adopt this methodology, as described on the project's website.
Size of the Library
To utilize any Moment.js function, the entire library must be loaded, contributing to a larger file size. This could impact performance, especially if only specific functions are needed.
Timezone
Moment.js has an extremely easy and powerful API for handling timezones. To use it, simply install the moment-timezone library:
For instance:
import moment from 'moment-timezone';
moment.tz('America/New_York').format('DD/MM/YYYY HH:mm'); // Defined Timezone
moment().format('DD/MM/YYYY HH:mm'); // Timezone from the device
Internationalization
To use internationalization in Moment.js you need the locale method and you can chain with another method, like the to method:
import moment from 'moment';
const resultInPortuguese = moment(new Date(2024, 0, 1)) // Object date
.locale('pt-BR') // Language: Brazilian Portuguese
.to([2024, 7, 1]);
const resultInEnglish = moment(new Date(2024, 0, 1)) // Object date
.locale('en-US') // Language: US English
.to([2024, 7, 1]);
console.log(resultInPortuguese); // Result: 'em 7 meses'
console.log(resultInEnglish); // Result: 'in 7 months'
Actually, moment.js faces performance issues with internationalization due to its non-use of the native JavaScript Intl API. It relies on its implementation for formatting and i18n for text translation.
Maintenance
Moment.js, one of the most significant players in JavaScript date handling, is now considered legacy. It receives only bug fixes and is not recommended for use in new projects, as stated on the official project site:
Date-fns
Mutability
Date-fns operates on the paradigm of immutability, derived from functional programming. In other words, its methods do not make changes to previously instantiated objects; instead, they create new objects when necessary, avoiding side effects.
Size of the Library
Date-fns allows for selective imports, enabling the loading of only the necessary functions. This results in a smaller file size and potentially better performance.
Internationalization
Date-fns utilizes the native JavaScript Intl API for formatting, leading to better performance in internationalization compared to Moment.js.
To work with internationalization in date-fns, simply import the desired languages from "date-fns/locale" and apply them to the desired function:
import { formatDistance } from 'date-fns';
import { ptBR, enUS } from 'date-fns/locale';
const resultInPortuguese = formatDistance(
new Date(2024, 7, 1),
new Date(2024, 0, 1),
{ locale: ptBR } // Language: Brazilian Portuguese
);
const resultInEnglish = formatDistance(
new Date(2024, 7, 1),
new Date(2024, 0, 1),
{ locale: enUS } // Language: US English
);
console.log(resultInPortuguese); // Result: '7 meses'
console.log(resultInEnglish); // Result: '7 months'
Timezones
You can handle timezone in Date-fns with the same locale syntax of internationalization (to show date strings with some timezone) or using the library date-fns-tz for a more robust API.
Maintenance
Date-fns is actively maintained, receiving bug fixes and new features consistently, as indicated on the official website.
Luxon
Mutability
Luxon follows the immutability paradigm, ensuring that its methods do not modify existing objects and its chainable:
DateTime
.local(2024, 1, 10)
.plus({ months: 1 })
.toISODate()
Size of the Library
Luxon has a small footprint and provides flexibility by allowing selective imports of only the required functions.
Internationalization
Luxon uses the native JavaScript Intl API for formatting, contributing to better performance in internationalization.
DateTime.now()
.setLocale("pt-BR")
.toLocaleString(DateTime.DATE_FULL);
Timezones
Luxon offers excellent timezone manipulation capabilities, making it straightforward to work with different time zones.
DateTime.now()
.setZone("America/New_York")
.minus({ weeks: 1 })
.endOf("day")
.toISO();
Maintenance
- Created by some maintainers of Moment.js;
- Luxon is actively maintained, offering continuous bug fixes and updates.
Day.js
Mutability
Day.js was created to be compatible with the syntax of Moment.js, but it follows the immutability paradigm, ensuring that its methods do not modify existing objects, and its chainable, like Luxon:
dayjs()
.startOf('month')
.add(1, 'day')
.set('year', 2024)
.format('YYYY-MM-DD HH:mm:ss');
Size of the Library
Day.js has a small footprint and provides flexibility by allowing selective imports of only the required functions.
Internationalization
Day.js uses the native Intl API for formatting and i18n for translation:
import 'dayjs/locale/pt-BR'
dayjs().locale('pt-BR').format()
Timezones
Day.js offers excellent timezone manipulation capabilities by using timezone and utc plugins:
import dayjs from 'dayjs';
import timezone from 'dayjs/plugin/timezone';
import utc from 'dayjs/plugin/utc';
dayjs.extend(timezone);
dayjs.extend(utc);
console.log(dayjs().tz('America/New_York').format('DD/MM/YYYY HH:mm'));
Maintenance
Day.js is actively maintained, offering continuous bug fixes and updates.
Conclusion
In summary, Moment.js, Date-fns, Luxon and Day.js have their strengths and weaknesses. Moment.js, despite its legacy status, offers a powerful API for handling timezones but falls short in terms of maintenance, mutability and size.
Date-fns, while actively maintained and immutable, it hasn't a robust API to handle timezones and has a smaller user base compared to Moment.js.
Luxon and Day.js keeps the same syntax from moment.js, but with better maintenance, size and immutability. The choice between them depends on the specific needs of the project.
To summarize the strenghts and weaknesses I created this table to facilitate your decision:
- 🌤 = Good
- ☁ = Neutral
- ⛈ = Bad
Thanks for reading!
References
Official documentation of Date-fns
Official documentation of Moment.js
Official documentation of Luxon
Official documentation of Day.js
https://www.youtube.com/watch?v=4ygUUFjQVqA